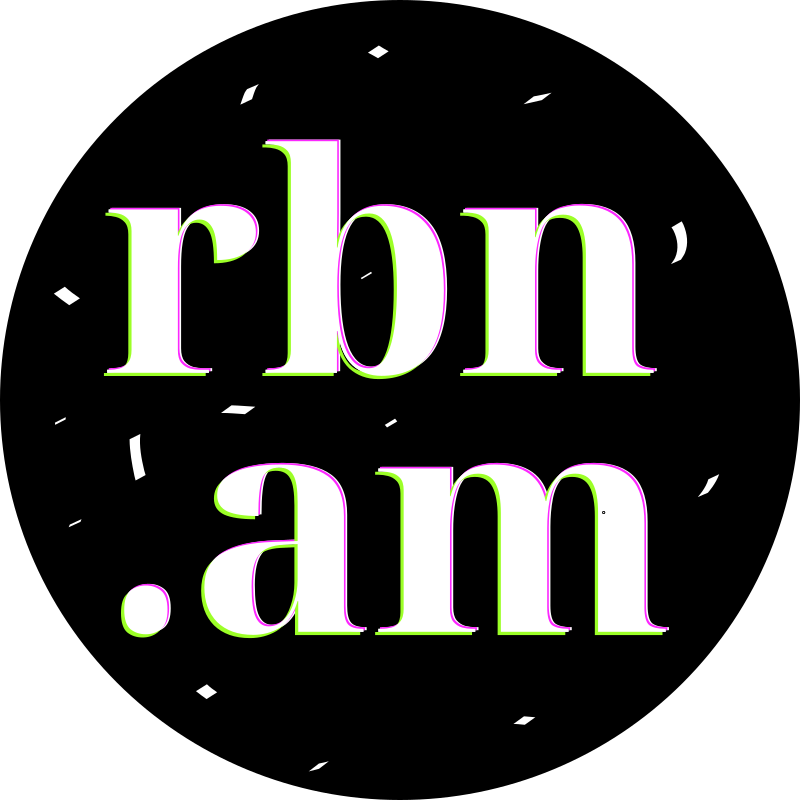
Ruben's Blog | Ռուբենի Բլոգը
🔙 ..Testing email sending with Jest, nodemailer, Node and TypeScript 📩
Ever since I started to do Test-driven development(TDD) I am encountered various issues regarding which I cannot find definitive solutions, so I will write my own.
Last time, I couldn’t make nodemailer to work with jest.
So imagine you have this REST API endpoint under /api/send-email
you want to test.
import nodemailer from 'nodemailer';
const transporter = nodemailer.createTransport({
host: 'smtp.host.com',
port: 465,
secure: true,
auth: {
user: 'REPLACE-WITH-YOUR-ALIAS@YOURDOMAIN.COM',
pass: 'REPLACE-WITH-YOUR-GENERATED-PASSWORD',
},
});
const sendEmail = async (req: Request, res: Response) => {
const email = 'some-email@mail.com';
try {
await transporter.sendMail({
from: `"Test email content" <${process.env.EMAIL_URL}>`,
to: email,
subject: 'Hello from test email.',
text: `This is a test email, thank you.`,
html: `
This is a test email,
<br>
thank you.
`,
});
res.status(200).send({ message: 'Please check your email.' });
} catch (err) {
res.status(400).send({ error: { message: 'Something went wrong.' } });
}
};
Now let’s test it with Jest.
import { htmlToText } from 'html-to-text'; // in order to test html content
// for some js reason this doesn't work with let
var sendMailMock: jest.Mock;
// mocking nodemailer
jest.mock('nodemailer', () => {
return {
__esModule: true, // this line makes it work!
default: {
createTransport: () => {
// be sure to use promise
sendMailMock = jest.fn((mail: Mail) => Promise.resolve(mail));
return { sendMail: sendMailMock };
},
},
};
});
beforeEach(() => {
sendMailMock.mockClear();
});
describe('{email test}', () => {
test('POST /api/send-email should send an email', async () => {
const email = 'some-email@mail.com'
const body = {};
const response = await request(app)
.post('/api/send-email')
.send(body);
expect(response.status).toBe(200);
// checking the argument that was passed to the sendMail function
const [args] = sendMailMock.mock.calls[0] as any;
expect(sendMailMock).toHaveBeenCalled();
expect(args.to).toBe(email);
expect(args.subject).toBe('Hello from test email.');
const htmlText = htmlToText(args.html);
expect(htmlText).not.toBe('');
const emailContaingTexts = [
'This is a test email,',
'thank you.'
];
emailContaingTexts.forEach((emailContaingText) =>
expect(htmlText).toContain(emailContaingText),
);
});
});
This way you can test any aspect of email sending.
Please give me feedback, comment on whatever you think about my blog post, and help me improve. ❤️
Blog Menu
- 💼 hire my consultancy
- ℹ️ about me
- 📰 rss
- 🛸 a young blog
- 📝 blog
- 2024-12-29
Then the Journey Is Not About Winning 🏆️
Winning is the target, but the journey is never about winning. You cannot be sure the path is correct. Even with guidance, there is a chance it’...
- 2024-12-28
Free Consultancy 💆🏻
Nobody buys it. When I provide it everyone is happy. Why? Maybe people don’t understand the offer, which is completely understandable. What I of...
- 2024-12-28
The Algorithm of the Dressings 🥬➕🥕➕❓️🟰🥗
Martun said he read many books about healthy food and didn’t become healthy. I agree, that reading and doing is very different. But I think that...
- 2024-12-25
Powered.community Meetup 🤝🏻
Well, it happened. The food was sushi, and people came with friends, some just checking in. I was so glad to see so many people I know and don’t...
- 2024-12-23
5 Books to Read Before 2025 to Transform Your Life 📚️
People are not computers, though we share traits such as short and long-term memory or processing power. You cannot install a “software” o...
- 2024-12-21
Ikigai 🥚
Opportunities come and go, but sometimes there is THAT opportunity. It makes my heart sing, puts me in sync with what I live for, and creates purpose ...
- 2024-12-19
Piece Is Pressure
We are not in a hot war right now, but it’s always over the corner. Many countries are in hot wars at this moment, what do they tell us? What wo...
- 2024-12-17
I Met My Mentor 🦉
My mentor probably doesn’t know she is my mentor. And I understood some things. I did a lot of analysis. She told me about the start stop and co...
- 2024-12-17
I'm Still Learning 🐇
I find myself suggesting the right solutions, right for me. And when I see that the pattern doesn’t match, I get sad. It’s hard to acknowl...
- 2024-12-13
Rushing the Process Doesn't Help ⌛️
I’ve started too late, because of that I have more knowledge from the field. I have been learning these past 4 years, but starting something by ...
- See all...
- 🔗 links